スッキリシリーズの名著、「すっきりわかるサーブレット&JSP」のメインコンテンツはつぶやきアプリの作成(dokoTsubu)だ。今回はそれを終えた人の演習課題としておすすめ映画紹介サイトを作成する。
実行例
localhost:8080/movie/index.jspにアクセスすると以下のようにログインフォームが表示される
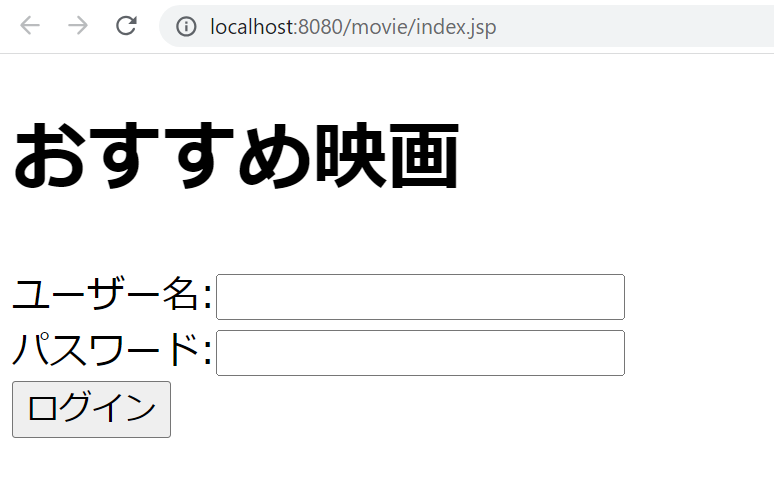
ユーザー名とパスワードを入力して、ログインをする。
今回は一律1234を正しいパスワードとする。
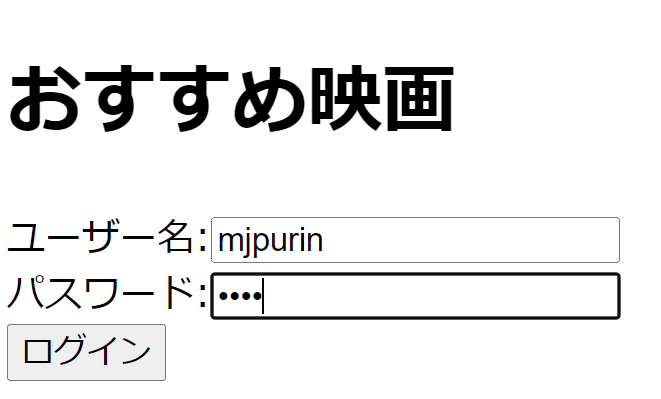
ログインに成功すると以下の画面が表示される
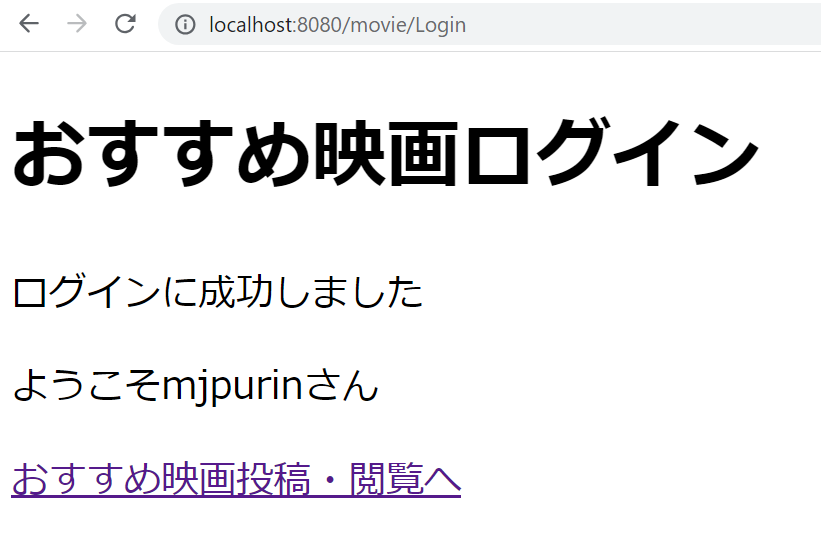
もし、間違ったパスワードを入力した場合以下の画面が表示される。
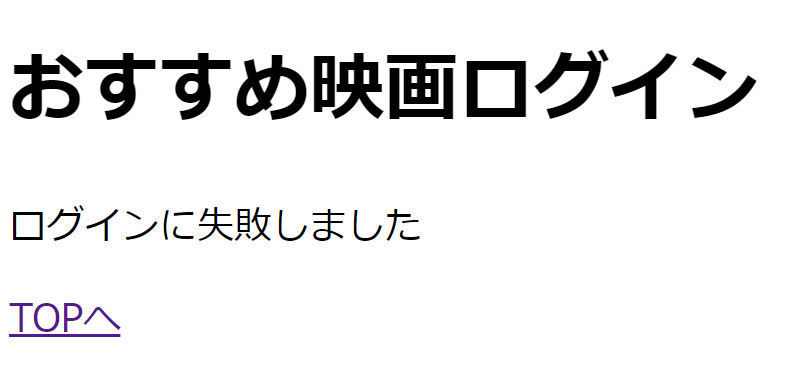
ログインに成功すると、投稿・閲覧のリンクが出てくるので押してみよう。
以下のようなページが表示される。
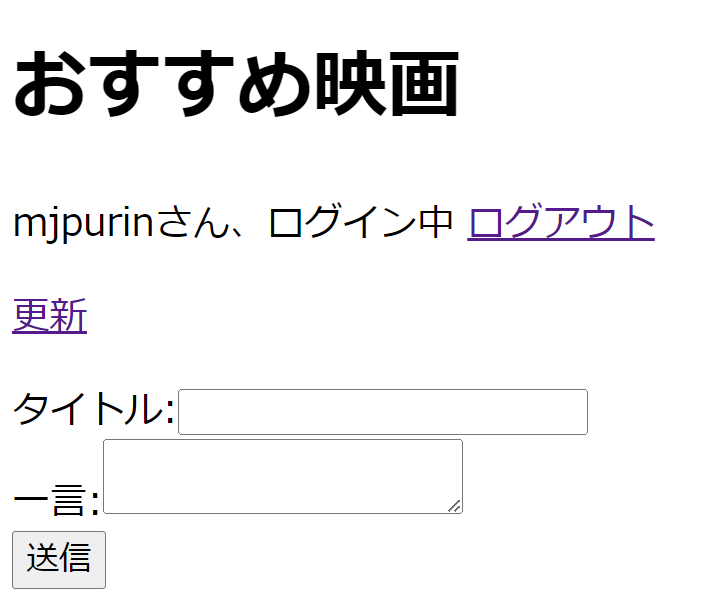
ここに自分のおすすめの映画タイトルと一言を記入する。
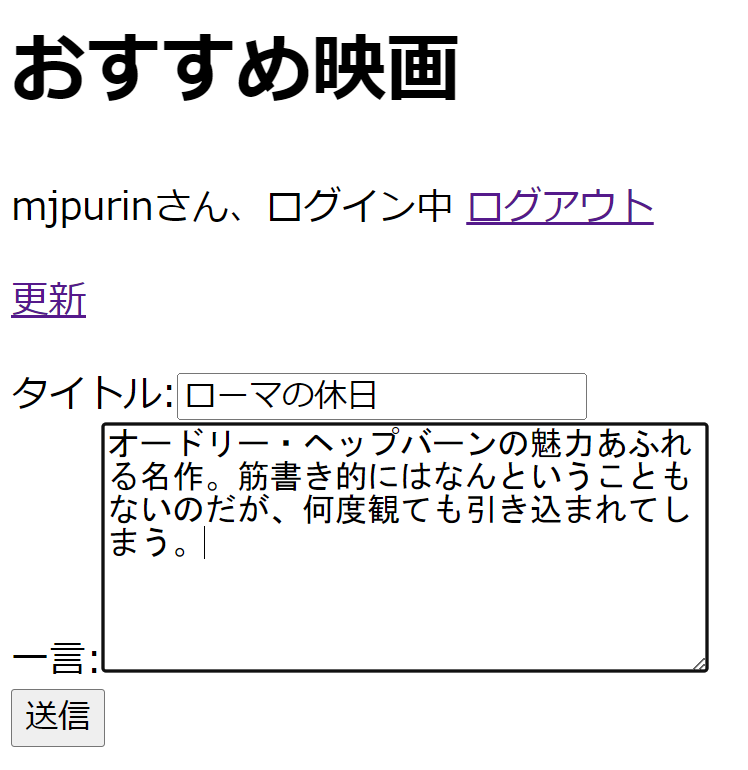
入力が終わったら送信ボタンを押す。
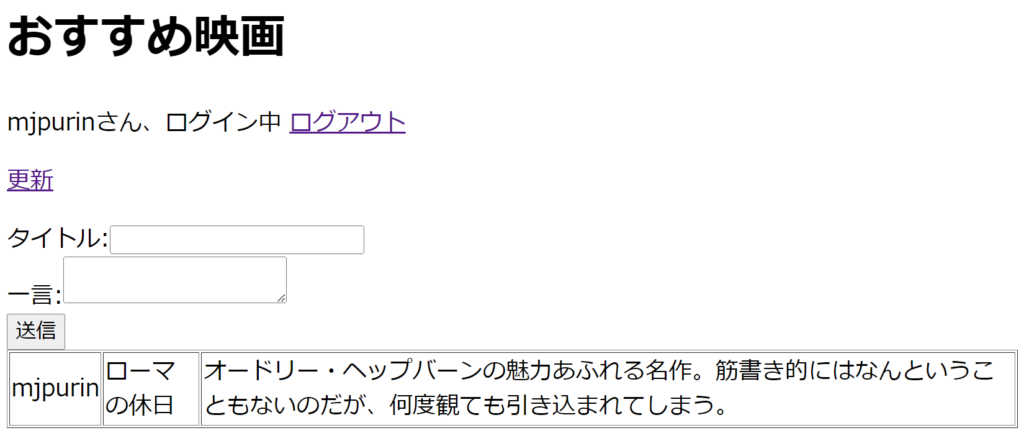
このように結果がテーブル表示される。続けて入力しよう。
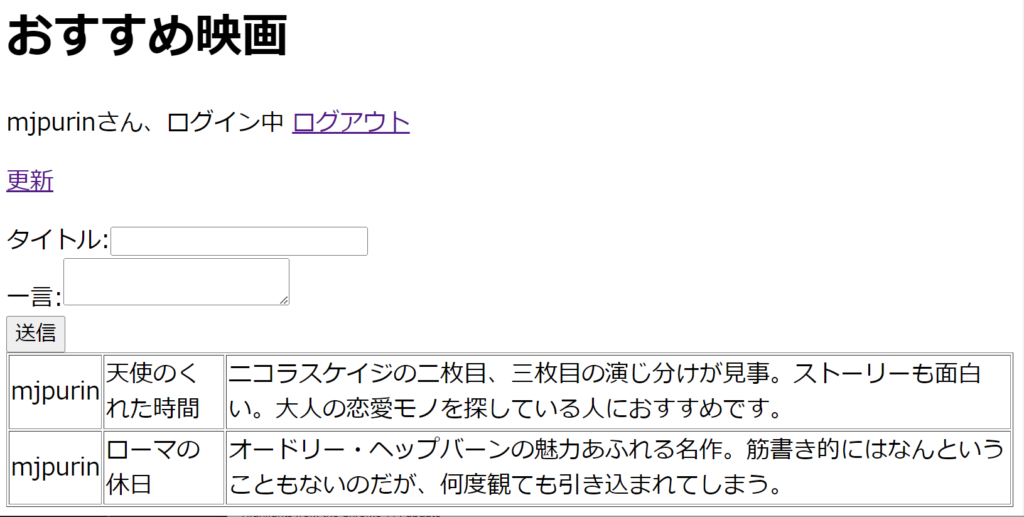
このように新しくいれたデータは上に表示される。
もし、データ入力際にタイトルを空にしたままま送信するとエラーメッセージが表示される。(一言の入力は任意とする)
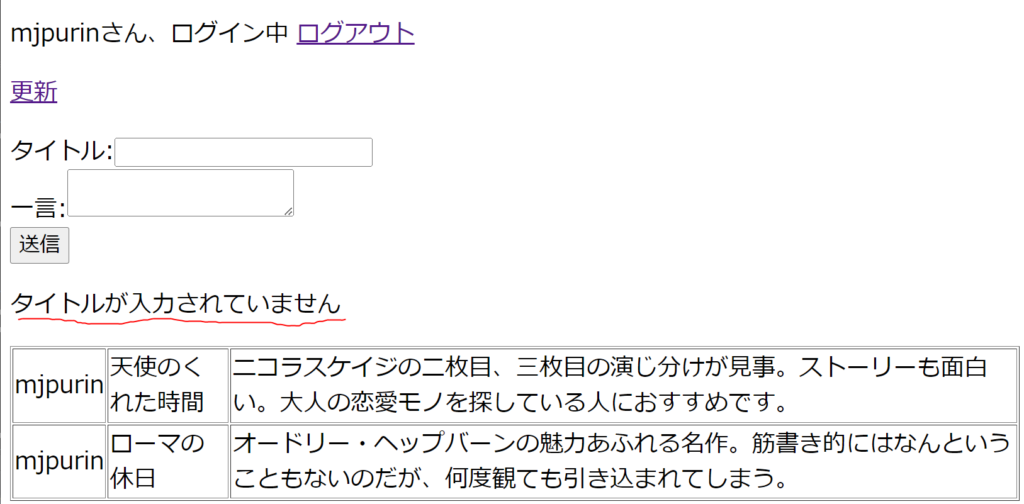
上部のログアウトのリンクを押すと以下の画面が表示される
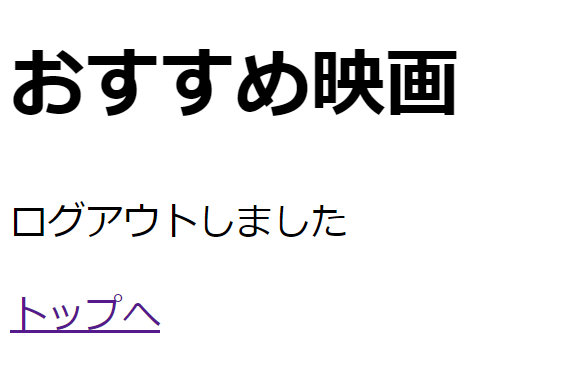
作例
1.新規動的Webプロジェクトからmovieという名前でプロジェクトを作成する。
最終的なファイル構成は以下。迷ったらすぐに参照すること
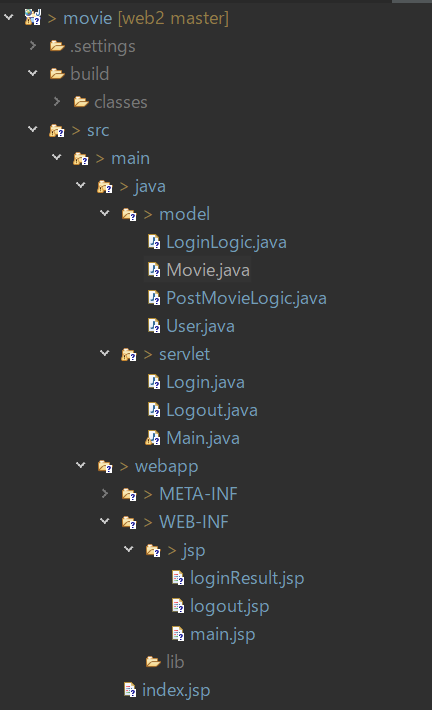
2.新規クラスからユーザーに関する情報を持つmodel.Userを以下のように作成する。
package model;
import java.io.Serializable;
public class User implements Serializable{
private String name;
private String pass;
public User() {}
public User(String name,String pass) {
this.name = name;
this.pass=pass;
}
public String getName() {
return name;
}
public String getPass() {
return pass;
}
}
modelパッケージ内に、以下のようにmodel.Movieクラスを作成する。
package model;
import java.io.Serializable;
public class Movie implements Serializable{
private String userName;
private String title;
private String comment;
public Movie() {}
public Movie(String userName,String title,String comment) {
this.userName = userName;
this.title=title;
this.comment=comment;
}
public String getUserName() {
return userName;
}
public String getTitle() {
return title;
}
public String getComment() {
return comment;
}
}
webappの直下にindex.jspを以下のように作成する。
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>おすすめ映画</title>
</head>
<body>
<h1>おすすめ映画</h1>
<form action = "Login" method="post">
ユーザー名:<input type="text" name="name"><br>
パスワード:<input type="password" name="pass"><br>
<input type="submit" value="ログイン">
</form>
</body>
</html>
ログインに関する処理を行うmodel.LoginLogic.javaを以下のように作成する。
package model;
public class LoginLogic {
public boolean execute(User user) {
if(user.getPass().equals("1234")) {
return true;
}
return false;
}
}
おすすめ映画の投稿に関する処理を行うモデルとして、model.PostMovieLogic.javaを以下のように作成する。
package model;
import java.util.List;
public class PostMovieLogic {
public void execute(Movie movie,List<Movie> movieList) {
movieList.add(0,movie);
}
}
ログインに関する処理を行うservlet.Login.javaを以下のように作成する
package servlet;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import model.LoginLogic;
import model.User;
@WebServlet("/Login")
public class Login extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
String name=request.getParameter("name");
String pass = request.getParameter("pass");
User user = new User(name,pass);
LoginLogic loginLogic = new LoginLogic();
boolean isLogin=loginLogic.execute(user);
if(isLogin) {
HttpSession session =request.getSession();
session.setAttribute("loginUser", user);
}
RequestDispatcher dispatcher=
request.getRequestDispatcher("WEB-INF/jsp/loginResult.jsp");
dispatcher.forward(request, response);
}
}
ログイン結果画面を出力するビューとして、WEB-INF/jsp/loginResult.jspを以下のように作成する。
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model.User" %>
<%
User loginUser = (User)session.getAttribute("loginUser");
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>おすすめ映画</title>
</head>
<body>
<h1>おすすめ映画ログイン</h1>
<%if (loginUser != null){ %>
<p>ログインに成功しました</p>
<p>ようこそ<%=loginUser.getName() %>さん</p>
<a href="Main">おすすめ映画投稿・閲覧へ </a>
<%}else{ %>
<p>ログインに失敗しました</p>
<a href="index.jsp">TOPへ</a>
<%} %>
</html>
メインの処理を行うコントローラをservlet.Main.javaとして以下のように作成する。
package servlet;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import model.Movie;
import model.PostMovieLogic;
import model.User;
@WebServlet("/Main")
public class Main extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
ServletContext application = this.getServletContext();
List<Movie> movieList = (List<Movie>) application.getAttribute("movieList");
if (movieList == null) {
movieList = new ArrayList<>();
application.setAttribute("movieList", movieList);
}
HttpSession session = request.getSession();
User loginUser = (User) session.getAttribute("loginUser");
if (loginUser == null) {
response.sendRedirect("index.jsp");
} else {
RequestDispatcher dispatcher = request.getRequestDispatcher("WEB-INF/jsp/main.jsp");
dispatcher.forward(request, response);
}
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
String title= request.getParameter("title");
String comment = request.getParameter("comment");
if(title != null && title.length() != 0) {
ServletContext application = this.getServletContext();
List<Movie> movieList =
(List<Movie>)application.getAttribute("movieList");
HttpSession session = request.getSession();
User loginUser = (User)session.getAttribute("loginUser");
Movie movie = new Movie(loginUser.getName(),title,comment);
PostMovieLogic postMovieLogic = new PostMovieLogic();
postMovieLogic.execute(movie, movieList);
application.setAttribute("movieList",movieList);
}else {
request.setAttribute("errorMsg","タイトルが入力されていません");
}
RequestDispatcher dispatcher =
request.getRequestDispatcher("WEB-INF/jsp/main.jsp");
dispatcher.forward(request, response);
}
}
メインのビューとして、WEB-INF/jsp/main.jspを以下のように作成する。
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import ="model.User,model.Movie,java.util.List" %>
<%
User loginUser = (User)session.getAttribute("loginUser");
List<Movie> movieList = (List<Movie>)application.getAttribute("movieList");
String errorMsg = (String)request.getAttribute("errorMsg");
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>おすすめ映画</title>
</head>
<body>
<h1>おすすめ映画</h1>
<p>
<%=loginUser.getName() %>さん、ログイン中
<a href="Logout">ログアウト</a>
</p>
<p><a href="Main">更新</a></p>
<form action ="Main" method="post">
タイトル:<input type="text" name="title"><br>
一言:<textarea name="comment"></textarea><br>
<input type="submit" value="送信">
</form>
<% if (errorMsg != null){ %>
<p><%=errorMsg %></p>
<%} %>
<% if (movieList.size()>0){ %>
<table border ="1">
<% for(Movie movie:movieList){ %>
<tr>
<td><%=movie.getUserName() %></td>
<td><%=movie.getTitle() %></td>
<td><%=movie.getComment() %></td>
</tr>
<%} %>
</table>
<%} %>
</body>
</html>
ログアウトに関するコントローラをservlet.Logout.javaとして以下のように作成する
package servlet;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
@WebServlet("/Logout")
public class Logout extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
HttpSession session = request.getSession();
session.invalidate();
RequestDispatcher dispatcher =
request.getRequestDispatcher("WEB-INF/jsp/logout.jsp");
dispatcher.forward(request, response);
}
}
ログアウトに関するビューをWEB-INF/jsp/logout.jspとして以下のように作成する。
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>おすすめ映画</title>
</head>
<body>
<h1>おすすめ映画</h1>
<p>ログアウトしました</p>
<a href="index.jsp">トップへ</a>
</body>
</html>
コメント