JavaScriptを使って以下の処理を作成してみよう。
仕様
実行すると以下のように表示される

フルーツの名前と価格を入れ登録ボタンを押す

下部にリストが表示され、入力欄が空になる

続いて入力しよう。今度はばなな100円を登録する
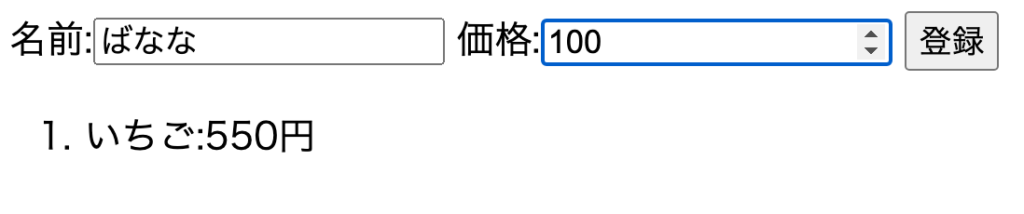
するとリストは価格降順に並ぶ
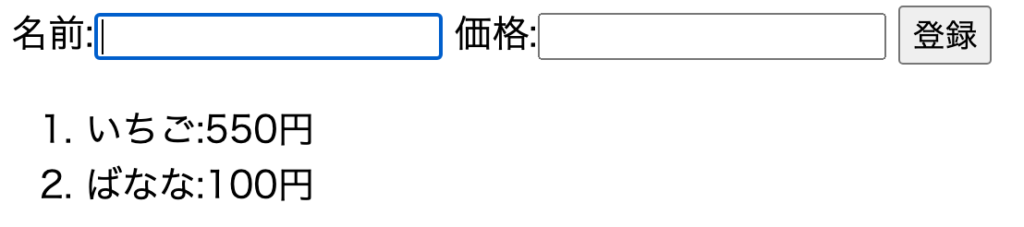
続いてメロン、1200円を登録すると。。。
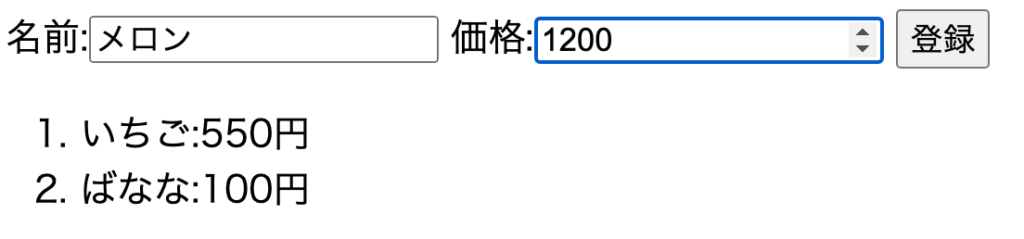
価格が1000円以上のフルーツは赤文字で表示される
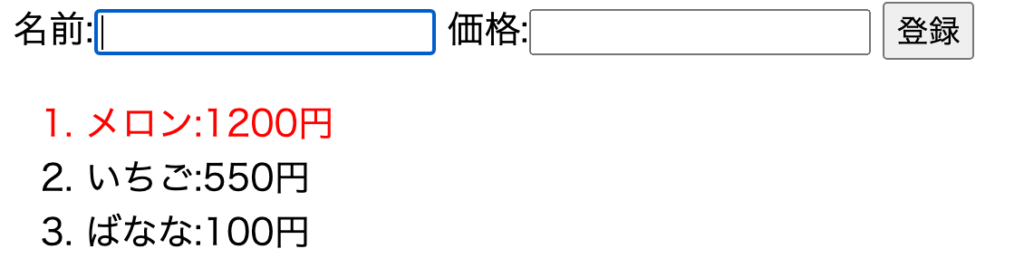
Let’s challenge!
では、このお題を作成してみよう。なお、データの永続化は考えなくてよい。
(ブラウザリロードで消えてよい)
作成
まずは以下のようにindex.htmlを作成する
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Test</title>
<style> .red{ color:red; } </style>
</head>
<body>
名前:<input type="text" id="name">
価格:<input type="number" id="price">
<button id="bt">登録</button>
<ol id="list"></ol>
<script src="main.js"></script>
</body>
</html>
続いてmain.jsを以下のように記述
'use strict';
document.addEventListener('DOMContentLoaded',()=>{
console.log('in');
class Fruits{
constructor(name,price){
this.name=name;
this.price=price;
}
showInfo(){
return `${this.name}:${this.price}円`;
}
}
const fruitsList=[];
//DOMの取得
const name=document.getElementById('name');
const price=document.getElementById('price');
const bt=document.getElementById('bt');
const ol=document.getElementById('list');
bt.addEventListener('click',()=>{
const fruitsName=name.value;
const fruitsPrice=parseInt(price.value);
fruitsList.push(new Fruits(fruitsName,fruitsPrice));
sortFruits();
ol.textContent=null;
for(let fruit of fruitsList){
const li=document.createElement('li');
li.textContent=fruit.showInfo();
if(fruit.price >= 1000){
li.classList.add('red');
}
ol.append(li);
}
name.value="";
price.value="";
name.focus();
});
const sortFruits=()=>{
for(let i=0;i<fruitsList.length-1;i++){
for(let j=i+1;j<fruitsList.length;j++){
if(fruitsList[i].price < fruitsList[j].price){
const fruit=fruitsList[i];
fruitsList[i]=fruitsList[j];
fruitsList[j]=fruit;
}
};
}
};
});
コメント