1回ダイスを振るのに300円かかる。1~6までのダイスの目をコンプリートするのにかかった回数と金額を表示するアプリを作成せよ。
今回はこのアプリをPHPを使って作成してみよう。データの保持にはセッションを使っていく。
フォルダ構成
フォルダ構成を確認しておこう。phpファイル自体は1枚だ。とてもシンプルな構成になっている。
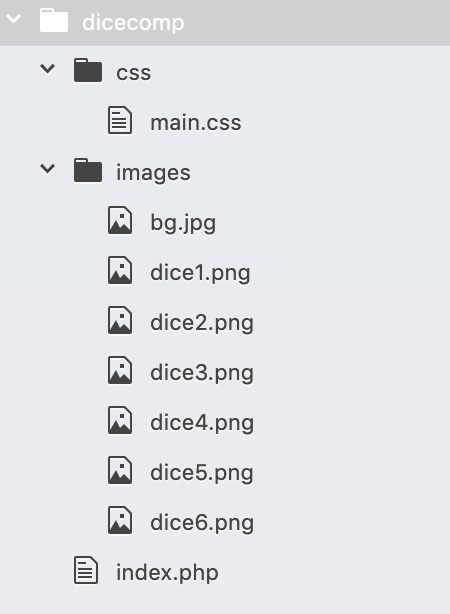
作成
1.dicecompフォルダを作成しその中にimagesフォルダ,cssフォルダを作成する。
2.以下からzipファイルをダウンロードし、展開したのち中に入っている画像7枚をimagesフォルダにいれる(フォルダ構成参照)
3.今回作成するのは以下のような見た目を持つゲームだ。
Diceボタンを押すとサイコロが振られ、その出目が上のリストから消えていく。
下には何回目かという情報とここまでにいくら使ったかが表示されている。
すべてコンプすることができればクリアだ。
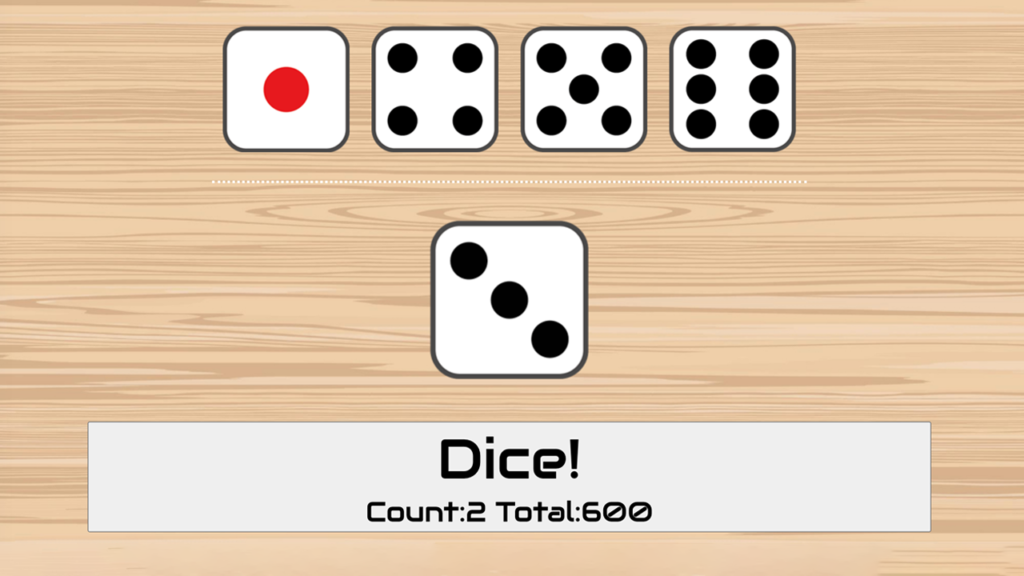
このゲームを作成するには何をセッションにいれればよいだろうか?その辺りを考えながらコーディングしていく。
index.phpの作成
4.index.phpを以下のように作成しよう。
<?php
session_start();
if(isset($_POST['bt'])){
if(in_array(0,$_SESSION['diceStates'])){
$index=rand(0,5);
for($i=0;$i<count($_SESSION['diceStates']);$i++){
if($_SESSION['diceStates'][$i]===1){
$_SESSION['diceStates'][$i]++;
}
}
$_SESSION['diceStates'][$index]++;
$_SESSION['count']++;
$_SESSION['total']+=300;
$_SESSION['nowDice']=$index+1;
$_SESSION['msg']="Count:{$_SESSION['count']} Total:{$_SESSION['total']}";
if(!in_array(0,$_SESSION['diceStates'])){
$_SESSION['msg'].=" Complete! Try again!";
}
}else{
$_SESSION=[];
}
}
$diceStates=isset($_SESSION['diceStates'])?$_SESSION['diceStates']:[0,0,0,0,0,0];
$count=isset($_SESSION['count'])?$_SESSION['count']:0;
$total=isset($_SESSION['total'])?$_SESSION['total']:0;
$nowDice=isset($_SESSION['nowDice'])?$_SESSION['nowDice']:0;
$msg=isset($_SESSION['msg'])?$_SESSION['msg']:"Push this Button";
$_SESSION['diceStates']=$diceStates;
$_SESSION['count']=$count;
$_SESSION['total']=$total;
$_SESSION['nowDice']=$nowDice;
$_SESSION['msg']=$msg;
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>DiceGame</title>
<link rel="preload" href="images/bg.png" as="image">
<!--google web fontの読み込み-->
<link rel="preconnect" href="https://fonts.gstatic.com">
<link href="https://fonts.googleapis.com/css2?family=Audiowide&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/main.css"/>
</head>
<body>
<div id="dices">
<?php for($i=0;$i<count($diceStates);$i++):?>
<?php if($diceStates[$i] <=1):?>
<img src="images/dice<?=$i+1?>.png" class="<?=$diceStates[$i]==1?"diceOut":""?>">
<?php endif;?>
<?php endfor;?>
</div>
<div id="dice">
<?php if($nowDice != 0):?>
<img src="images/dice<?=$nowDice?>.png" class="diceIn">
<?php endif;?>
</div>
<form method="post">
<button type="submit" name="bt">Dice!<span class="result"><?=$msg?></span></button>
</form>
</body>
</html>
ポイントはdiceStates配列だ。1~6の目に対して
0:一度も出ていない
1:初めて出た(diceOutクラスを付与して消すアニメーション)
2以上:過去に出た(非表示)
という状態を設定して表示の仕方を変えていく。
0が無くなったらコンプを意味する。
main.cssの作成
5.main.cssを以下のように作成する。
body {
background: url(../images/bg.jpg) #efcfa9;
background-size: cover;
height: 100vh;
display: flex;
flex-direction: column;
justify-content: space-around;
align-items: center;
}
#dices {
display: flex;
justify-content: space-around;
padding-bottom: 3vh;
border-bottom: 3px dotted white;
}
#dices img {
width: 12vw;
height: 12vw;
max-width: 170px;
margin: 2vmin;
}
#dice img {
/*最初は透明にして見えないようにしておく*/
opacity: 0;
}
button {
font-family: 'Audiowide', cursive;
width: 80vw;
font-size: 10vmin;
}
button:hover {
cursor: pointer;
}
button span {
font-size: 5vmin;
opacity: 0;
}
.diceHidden {
display: none;
}
.diceOut {
animation-name: diceOut;
animation-delay: 1.5s;
animation-duration: 2s;
animation-fill-mode: forwards;
}
@keyframes diceOut {
to{
width: 0;
margin: 0;
opacity: 0;
}
}
.diceIn {
animation-name: diceIn;
animation-delay: 0.5s;
animation-duration: 1s;
animation-fill-mode: forwards;
}
@keyframes diceIn {
from {
width:0;
height: 0;
opacity: 0;
transform: rotateX(0);
}
to {
width: 15vw;
height: 15vw;
opacity: 1;
transform: rotateX(360deg);
}
}
.result {
display: block;
animation-name: result;
animation-delay: 1.5s;
animation-duration: 1s;
animation-fill-mode: forwards;
}
@keyframes result {
from {
opacity:0;
}
to {
opacity: 1;
}
}
このHtml/CSSに関してはこちらの記事を参考にしてもらいたい。
完成
以上で完成だ。最短6回でのコンプを目指して遊んで見てほしい。
以下のリンクから実際に遊ぶことができる。
https://joytas.net/php/dicecomp/
コメント