mvcとセッションスコープ、リクエストスコープを使って野菜カートアプリを作成してみよう!
見た目の表示にはbootstrap4をしている。
実行例
実行するとフォームが表示される
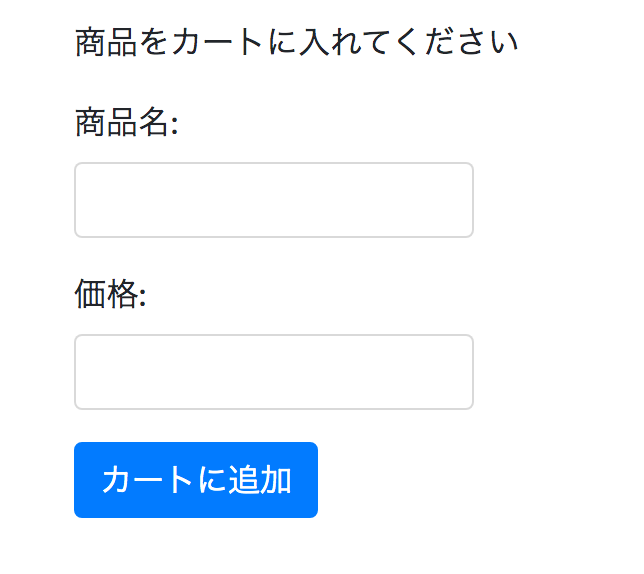
フォームに野菜の名前と価格を入れる。
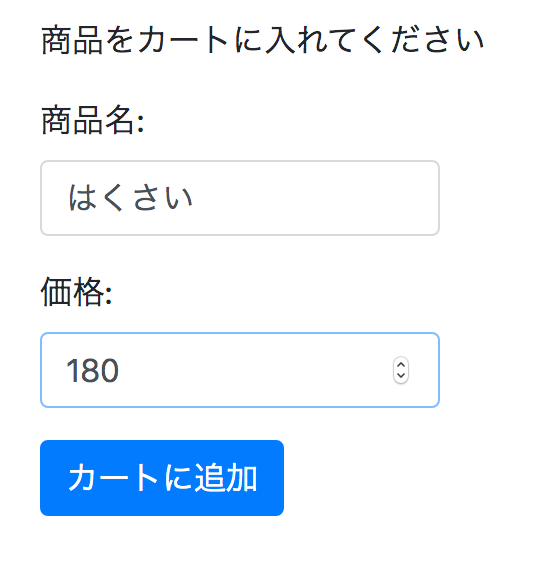
カートに追加ボタンを押すとカートに追加される
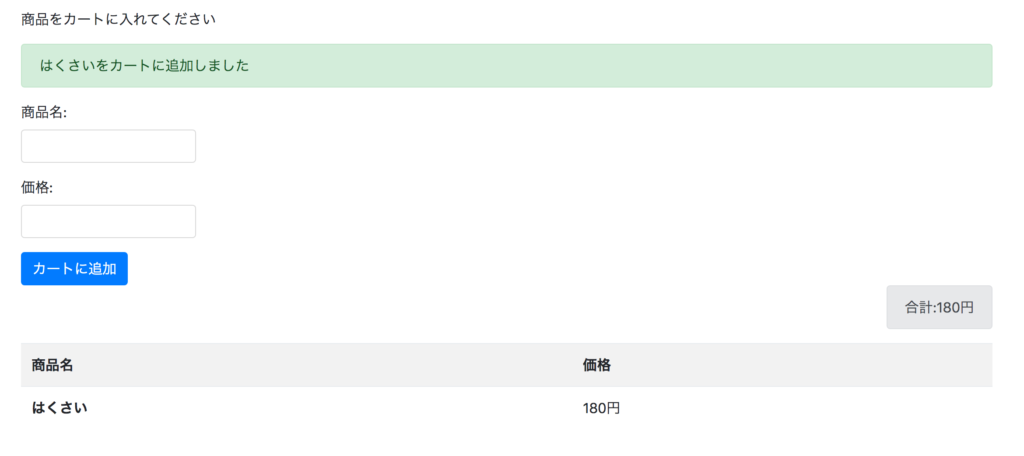
二つ目にトマトを追加した
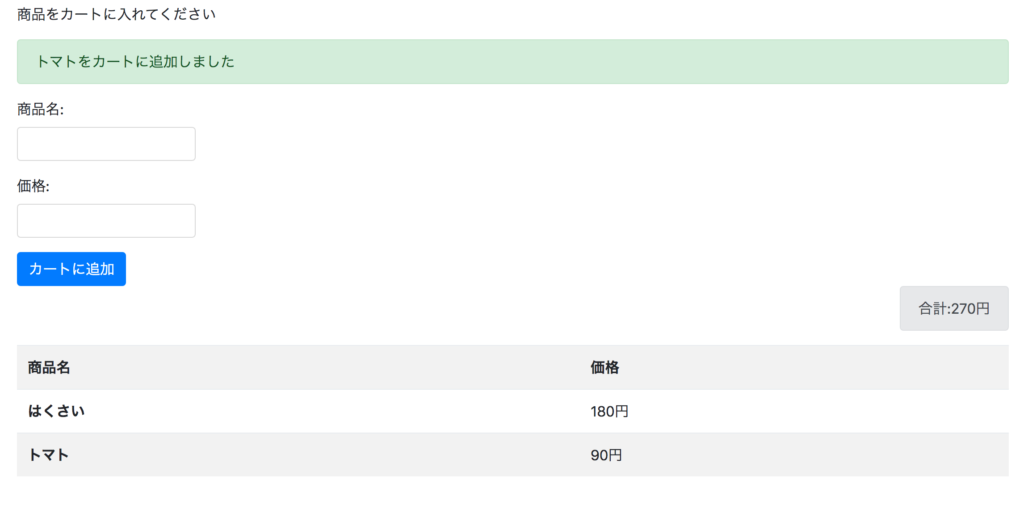
商品名や価格を空で送信するとエラーが表示される
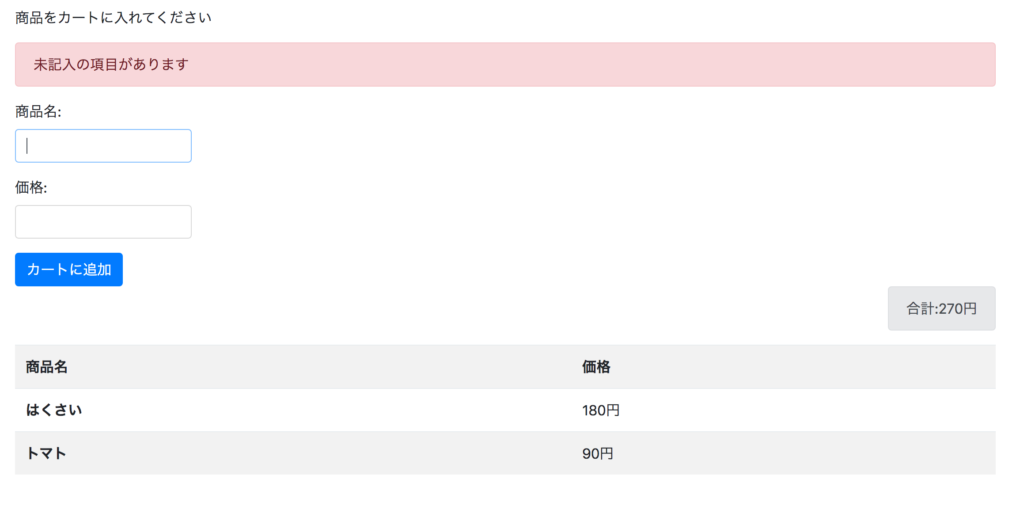
解答例
package model;
import java.io.Serializable;
public class Vegetable implements Serializable{
private String name;
private int price;
public Vegetable() {
super();
}
public Vegetable(String name, int price) {
super();
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
}
package model;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
public class Cart implements Serializable{
private List<Vegetable> list;
private int total;
public Cart() {
super();
list=new ArrayList<>();
total=0;
}
public List<Vegetable> getList() {
return list;
}
public void setList(List<Vegetable> list) {
this.list = list;
}
public int getTotal() {
return total;
}
public void setTotal(int total) {
this.total = total;
}
}
package model;
public class CartLogic {
public void execute(Cart cart,Vegetable vege) {
cart.getList().add(vege);
cart.setTotal(cart.getTotal()+vege.getPrice());
}
}
package controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import model.Cart;
import model.CartLogic;
import model.Vegetable;
@WebServlet("/Main")
public class Main extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
RequestDispatcher rd=request.getRequestDispatcher("/WEB-INF/view/cart.jsp");
rd.forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
String name=request.getParameter("name");
String price=request.getParameter("price");
if(name.isEmpty() || price.isEmpty()) {
request.setAttribute("err", "未記入の項目があります");
}else {
HttpSession session=request.getSession();
Cart cart=(Cart)session.getAttribute("cart");
if(cart==null) {
cart=new Cart();
}
Vegetable vege=new Vegetable(name,Integer.parseInt(price));
CartLogic logic=new CartLogic();
logic.execute(cart, vege);
session.setAttribute("cart", cart);
request.setAttribute("msg", vege.getName()+"をカートに追加しました");
}
doGet(request, response);
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8" import="model.*,java.util.*"%>
<%
Cart cart=(Cart)session.getAttribute("cart");
String err=(String)request.getAttribute("err");
String msg=(String)request.getAttribute("msg");
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8"/>
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-beta/css/bootstrap.min.css" integrity="sha384-/Y6pD6FV/Vv2HJnA6t+vslU6fwYXjCFtcEpHbNJ0lyAFsXTsjBbfaDjzALeQsN6M" crossorigin="anonymous">
<title>VegetableCart</title>
</head>
<body>
<div class="container" style="margin-top:20px;">
<p>商品をカートに入れてください</p>
<% if(err !=null){%>
<div class="alert alert-danger" role="alert">
<%=err %>
</div>
<%} %>
<% if(msg !=null){%>
<div class="alert alert-success" role="alert">
<%=msg %>
</div>
<%} %>
<form action="/vegecart/Main" method="post" >
<div class="form-group">
<label for="name">商品名:</label>
<input type="text" id="name" name="name" class="form-control" style="width:200px;">
</div>
<div class="form-group">
<label for="price">価格:</label>
<input type="number" id="price" name="price" class="form-control" style="width:200px;">
</div>
<button type="submit" class="btn btn-primary">カートに追加</button>
</form>
<%if(cart != null){%>
<div class="alert alert-secondary float-right" role="alert">
<%=String.format("合計:%,d円",cart.getTotal()) %>
</div>
<table class="table table-striped mt-4">
<tr><th>商品名</th><th>価格</th></tr>
<%for(Vegetable v:cart.getList()) {%>
<tr><th><%=v.getName() %></th><td><%=String.format("%,d円",v.getPrice()) %></td></tr>
<%} %>
</table>
<%} %>
</div>
</body>
</html>
コメント